Register Yourself to get hired soon:- Register Now
In Python, six data types are found:-
- Number
- String
- List
- Tuple
- Dictionary
- Set
In Python data, types are two types:-
1.Mutable type(Non- Changeable)
2. Immutable type(Changeable)
Mutable Data Type:-
The Data type can not be changed after being created once that is called Mutable Data types. these Data types are not changeable. Some of the mutable data types in Python are list, dictionary, set, and user-defined classes
Accenture Mass Hiring For Freshers:- Apply Now
Immutable Data Type:-
The Data type can be changed after being created once that is called Immutable Data types. these Data types are changeable. Some of the immutable data types are int, float, decimal, bool, string, tuple, and range
Number Data Type:-
int, float, double, long, long double, and numbers data types, these are mutable types.

String Data Type:-
A string can be written in single as well as double-quotes. Python treat both single and double quotes are stored as individual characters in a contiguous memory location.
Contiguous:- Continous memory address given to string.
How to define String:-

indexing / slicing of string:
str =”W3hiring”
0 1 2 3 4 ———-Forward Indexing
-5 -4 -3 -2 -1 ————Backward indexing

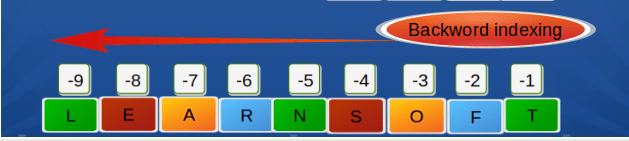
String Concatination Operator(+) :-
The concatenation operator will add two strings.
print("W3" + "hiring") output:- W3hiring
String Replication Operator ( * ):-
String replication operator will print the same word multiple times. if we multiple a word with a number.
print(5*"w3hiring") Output:- w3hiringw3hiringw3hiringw3hiringw3hiring
We can see in the above code we use a replication operator in between a word and a number. In the output, we got the same word multiple times as the number we used to multiply.
Note:- Slicing can only work on more than one value or group of values.
Slice Notation:-
A string slice can be defined as a substring which is the part of the string.


Explanation: In the Above code we stored a string in a variable named str. After that, we perform slicing on that string. we can write color inside pair of the square bracket if we want to print a full string. Or another way to print a string is indexing. we can use an index of string to slice a peculiar string. suppose that we have a string str=”w3hiring”. the length of the string is 8 and the index is 0-7. if we want to print the first two characters of the string we can write str[0:2]. it will print w3 in the terminal as Output.
String Function and methods:-
- Capitalize()
- Count()
- Endswith()
- find()
- index()
- isalnum()
- isalpha()
- isdigit()
- islower()
- isupper()
- isspace()
- upper()
- startswith()
- swapcase()
- tstrip()
- lstrip()
- rstrip()
Capitalize():
it capitalizes the first character of the string.
print('w3hiring'.capitalize()) #Output:- W3hiring
Sometimes we want to make the first character of word capital. like the name of the person. For Example, My name is ‘aamir’ and I want that the first character of the name should be capital automatically. we can use the capital for that work. it will do it automatically ( ‘aamir’.capitalize() —> Aamir)
Count(string, begin(optional), end(optional)):
Counts no of times substring occurs in a string between begin and end index.

there are three attributes given inside the count function sequentially substring, begin index, and end index. e is a substring, begin index is 2 and end index is 9 in the third line of code and we got 1 as result. We can see that the length of the string is 18. and the substring from index 2 to 9 is ‘lcome t’. Now we have to find that how many times a substring occurs inside this substring. we can see there is only one e inside substring. so we got result as 1. because it occurs once in the substring.
Endswith(string):-
Returns a boolean value if the string terminates with given suffices between beginning and end.
str="Welcome to w3hiring" substr1="w3hiring" substr2="to" substr3="of" print(str.endswith(substr1)) # Output : True print(str.endswith(substr2,2,16)) #Output :- False print(str.endswith(substr3,2,19)) #Output :- False print(str.endswith(substr3)) #Output :- False
In the above code, we have one string and three substrings. we have to find that ‘is our substring at the end or not‘. if our substring is at the end of the string it will return true otherwise false. Endswith function takes three attributes sequentially substring, begin index, end index. print(str.endswith(substr1)) will return True. As we can see our string is “Welcome to w3hiring” and w3hiring is at the end of the string. all other will return False.
Find(string):-
It returns the index value of the string where the substring is found between begin index and end index.
str= "welcome to w3hiring" substr1="come" substr2="to" print(str.find(substr1)) #Output : 3 print(str.find(substr2)) #Output: 8 print(str.find(substr1,3,10)) #Output: 3 print(str.find(substr2,19)) #Outpur: -1(by default)
Index(string):-
it works the same as find() except it raises an exception if the string is not found.
str="Welcome to w3hiring" substr2="to" print(str.index(substr2,20))
Note:- Above code will raise an exception. Because index 20 is not present.
Isalnum():
It returns true if no and character is in the string otherwise false.
Isalpha():
It returns True if the alphabets are there in the whole string.
isdigit():-
it returns True if characters are digit and there is at least one character, otherwise false.
islower():
It returns True if the Characters of a string are in lower case, otherwise false.
Defference between Function and Method.
Function:- Function can be directly called.
Method:- Methods are called by creating objects with the dot operator.
Isupper():
It returns false if characters of a string are in upper case, otherwise false.
Isspace():
The Spacing should have white space . there should be no character in it.
Lower():
Converts all the characters of a string to lower case.
Startswith(string):-
Returns a boolean value if the string starts with a given string between begin and end.
str="Hello W3hiring" print(str.startswith('Hello')) #Output: True str2="Welcome to W3hiring" print(str.startswith('come')) #Output: False
Swapcase():
Convert case of all string characters in a string.
str="Hello W3hiring" print(str.swapcase()) #Output:- hELLO w3HIRING str2="Welcome to W3hiring" print(str2.swapcase()) #Output:- wELCOME TO w3HIRING
Strip():
tstrip:- Returns all leading whitespace of a string. It can also be used to remove a particular character from leading.
str=" Hello Python" print(str.tstrip()) #Hello Python str2=" @@@Welcome to W3hiring" print(str2.tstrip("@")) #Welcome to W3hiring
Rstrip:- Returns all trailing whitespace of a string. It can also be used to remove a particular character from trailing.
str ="Hello Python3" print(str.rstrip()) #Output: Hello Python3 str2 = " @welcome to w3hiring!!" print(str2.rstrip("!")) #@welcome to w3hiring
We hop you have understood the concept of datatypes in Python. Thanks for visiting our website.