Register Yourself to get hired soon:- Register Now
Hy Guys In this article we will learn two main data types of python Tuples and dictionaries. we will learn with examples and explanations. if you are a beginner then don’t worry I will explain every line of code.
What is A Tuple?.
A Tuple is a sequence of immutable objects, therefore the tuple can not be changed the objects are enclosed within parenthesis and separated by a comma.
A tuple is similar to a list only the difference is that list is between parentheses and a list has mutable objects whereas a tuple has immutable objects. If parenthesis is not given with a sequence, it is by default treated as a tuple.
Advantage of tuples-
- Processing of tuples are faster than lists.
- It makes the data safe as tuples are immutable. hence can not be changed.
data1=(1,2,3,4) data2=('x','y','z') data3=(10,20,'aman',34.5) data4="a",10,16.5 print(data1[0]) #(1) print(data1[0:2]) #(1,2) print(data2[-3:-1]) #('x','y') print(data1[0:]) #(1,2,3,4) print(data2[:2]) #('x','y') print(data3[-4:-1]) #(10,20,'aman') print(data4[0:]) # "a",10,16.5
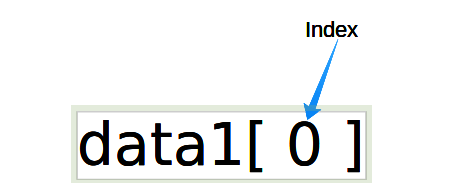
Let’s Understand what we have done in the above code. we will use the slicing method to perform operations on tuples so please read the previous article to learn slicing we have written valuable content on slicing in the previous article. first of all, we store three tuples in three different variables named data1, data2, data3. we stored numbers in data1 in a sequence and character in data2 and then we store random data in data3 in the form of a tuple. Now if we want to perform slicing on them or we want to print something, we can use the above method. we write data1[0] it will print the data from index 0 in data1. here colon is used for range. suppose we want to print 1,2 at a time we can use data1[0:2] it will print the value of the index 0,1 of data1. and in the next line, we print x,y using data2[-3:-1]. we used backword slicing here to print the characters x,y. we print the value for index -3,-2 only. if we write data2[0:] it will print all elements of the tuple.
For Example
Accenture Mass Hiring For Freshers:- Apply Now
Forward slicing
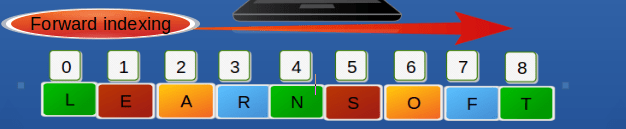
Backward Slicing:
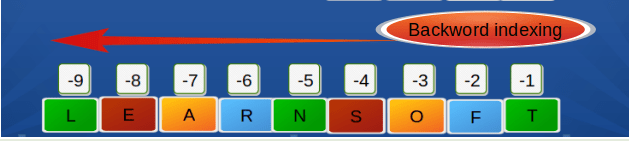
Adding tuple:-
Tuple can be added by using the concatenation operator (+) to join two tuples.
data1=(1,2,3,4) data2=('x','y','z') data3=data1+data2 print(data3) #Output:- (1,2,3,4,'x','y','z')
we use two variables to store two different tuples name data1 and data2. and then we concatenate both tuples using the + operator. it will place the data of the second tuple after the first tuple’s data.it will never add value. even if we fill both tuples with a number it will never add and it will perform the same.
For Example: data1=(1,2,3,4) , data2=(5,6,7,8) , data3=data1+data2, data3=data1+data2. if we print the value of data3 it will print (1, 2, 3, 4, 5, 6, 7, 8). it will add them horizontally.
For Example
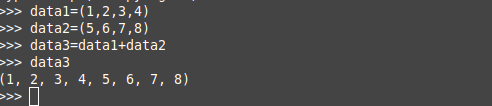
Replicating tuple:-
Replicating means repeating, it can be performed by using the ‘*’ operator a specific number of times.
tuple1=(10,20,30) tuple2=(40,50,60) print(tuple1*2) print(tuple2*3) #Output:- (20,40,60) (120,150,180)
Replicating the operator is nothing but multiple. this operator is used to multiply something with a number. like we store two tuples then we multiply the first tuple with number 2 and the second tuple with number 3. it will multiply every element of tuple with 2 in tuple1 and 3 in tuple2. but if we multiple characters with numbers it will print the character multiple times as per number.
For Example:-
print("hello"*5) #output:- hellohellohellohellohello
we multiply the “hello” string with 5. we got it 5 times as result.
Tuple Slicing:-
A subpart of a tuple can be retrieved on the basis of an index this subpart is known as slicing.
Updating Elements in a list:
Element of the tuple can not be updated this is due to the fact that tuples are immutable whereas the tuple can be used to form a new tuple.
tuple1=(10,20,30,40) tuple1[0]=100 print(tuple1) #Output :- Not possible(Immutable)
As we know tuple is immutable. so we can’t update the data of tuple. if we try to update a tuple we will get an error.
Creating a New Tuple from existing tuple:-
tuple1=(10,20,30) tuple2=(40,50,60) tuple3=tuple1+tuple2 #Output (10, 20, 30, 40, 50, 60)
we can create a new tuple from existing tuples using the concatenation operator (+). it will append all the elements of tuple2 after the elements of tuple1. And store all the data in tuple3 then print all the elements in the sequence format of the tuple.
Deleting element from Tuple:-
Deleting an individual Element from a tuple is not supported however the whole of the tuple can be deleted using the del statement.
tuple=(10,20,"Aamir",30.5,'a') print(tuple) del tuple print(tuple) #Output:- tuple Not Defined.
there is a keyword del. by using the del keyword we can delete any tuple. as we delete our tuple and then try to print it again it is showing tuple not defined. but before using the del keyword. it was accessible. but there is a twist in the del keyword if we want to delete a particular element from a tuple, we can’t. it will not allow us to delete an element from the tuple. because the tuple is immutable.
Functions Of Tuple:-
min(tuple)
Retrieve the min value from a tuple.
tuple=(10,20,30,40) print(min(tuple)) #Output:- 10
if we want to print the minimum element in a tuple we can use the min () function. it can retrieve the minimum element from a tuple. we should give the credit to python. who makes coding easy for us. we don’t need to see minimum element manually we can simply function and it will return the minimum element as result.
Max(tuple):
Returns the max value from the tuple.
tuple=(10,20,30,40) print(max(tuple)) #Output:- 40
As we use the minimum function we can use the max function to find the maximum element from a tuple. we don’t need to find the maximum element manually.,
len(tuple):
Returns the length of a tuple. len is a function of the tuple which is used to find the length of the tuple.
tuple=(10,20,30,40) print(len(tuple)) #Output:- 4